Using the Objectives Page of the Journal
While not necessary to the game itself, the Objectives page of the journal adds functionality and is an easy way for the player to keep track of what he's trying to do. It is best used to boil away the fat from the journal entries and other sources of vast information that are present in the world, so that the player also has a set of clear-cut goals to work toward.
How Objectives Work in Undying
It is not necessary to read this next paragraph to be able to do the tutorial here, but it does provide useful background information.
In Undying, objectives are static string variables that are part of the AeonsPlayer class. In other words, they are written directly into the properties of your PlayerPawn. Unfortunately, the main game's objectives are what have been written into the class by default. Therefore, something will need to be created that rewrites them with a set of custom objectives.
Making a New Objectives List
Make a subclass of Info from the actor class browser. Give it a unique class name, and give it a package name dependent on your needs. (Also note that there are many similarities between setting this up and creating a journal entry.)
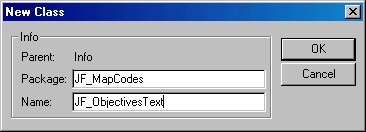
In the script editing window, copy this next piece of code and paste it underneath the class declaration of the new class you're creating.
var () string ObjectivesText[100];
var byte i;
function PreBeginPlay()
{
Tag = LevelBegin;
}
function Trigger(actor Other,Pawn EventInstigator)
{
ForEach AllActors(class 'AeonsPlayer', AP)
{
break;
}
for (i=0; i<=99; i++)
{
AP.ObjectivesText[i]=Self.ObjectivesText[i];
}
}
Though it's not really necessary, I would also encourage you to select a different icon for when you use the custom class in the editor. I personally prefer the document icon from the journal. This can be accomplished by selecting an icon in the texture browser, and then clicking on "Use" for the Texture variable of the actor's display properties. Once that's done, save the script package.
Now you'll need the use of an .int file. If you don't already have one with the same name as the script package itself, create it. All custom .int files for Undying should start with the following five lines of text and a blank line before any other data is added:
[General]
Start=Undying (Starting)
Exit=Undying (Exiting)
Run=Undying (Running)
Product=Undying
In the .int file, create the entry for your custom class. To show you part of what it looks like, I'll paste in a few of the main game's objective lines as examples of objectives.
[JF_ObjectivesText]
ObjectivesText[0]=
ObjectivesText[1]=17,Meet with your old friend Jeremiah Covenant.
ObjectivesText[2]=52,Investigate the noise downstairs.
ObjectivesText[3]=74,Investigate the Covenant family curse.
ObjectivesText[4]=72,Find Lizbeth Covenant's room.
ObjectivesText[5]=837,-THE LIZBETH QUEST-
ObjectivesText[6]=844,Kill Lizbeth Covenant.
ObjectivesText[7]=299,Enter gardens through kitchen.
ObjectivesText[8]=116,Find east wing key.
Points of interest
- You cannot have any more than 99 objectives. However, this will probably not be a problem, as the main game only used about 80 objectives. If necessary, you could create another ObjectivesText class with different variables that later maps use, but 99 objectives should be enough for anyone.
- You must always leave ObjectivesText[0] blank. Due to the nature of how objectives are added and removed from the player's journal, this particular variable should never be used. I will explain this later.
- You will need to precede each objective with a unique positive integer. These determine a list order for the objectives. These numbers are meaningless when the player has only a single objective on his objectives page, but they are used to organize objectives when the player has two or more at once. The active objectives will be displayed on the objectives page in descending order according to these numbers. That is why "-THE LIZBETH QUEST-" has a number higher than all the objective numbers that happen during the course of that part of the game.
Implementing your custom objectives list
To finish this tutorial, just place one of these actors in each level that uses this objectives list. Since all the pertinent data is stored in the actor's .int entry, then nothing else needs to be done. The actor will automatically change its tag to "LevelBegin" at the start of the map. This tag name is reserved by the game specifically for being triggered as a level starts, so the default objectives will change back to your custom ones immediately as each level is entered. Now all you need is the knowledge of how to add and remove objectives as you see fit.
How to add and remove objectives
There are two ways to add and remove objectives from a player's journal, and both of them use something called an "objective string". It is a string variable containing a list of numbers that facilitates this process. A sample objectives string might be: "-4 -5 +9 +10 -11". This means that objectives numbered 4, 5, and 11 are removed from the player's book, and 9 and 10 are added. (Of course, most objective strings will only contain one or two numbers, rather than the five numbers in this one.) This is why objective number zero should never be used; zero can be either positive or negative, which makes parsing of the objective string turn out a bit strange if zero is present.
The numbers in the objective string can go in any order, as long as a space is between each of them. These numbers represent the objectives' positions in the ObjectivesText array, not the numerical tags that the objectives have.
An objectives string can be used in either of two places:
- A journal entry. Journal entries have a variable simply called "Objectives" that this string can be entered into. It can be included in the journal entry's .int file along with the title and main text. When a player receives the journal entry, his Objectives page will be altered as well.
- An ObjectivesTrigger. An ObjectivesTrigger is actually a subclass of Info, rather than Trigger as the class name seems to imply. Place one in your map, input the objective string into its Objectives variable, and give the actor a unique tag. When a player triggers it in the game, his Objectives page will be altered to reflect the changes in active objectives.